Need a Fresh Start? How to Restart ServiceNow Workflows Programmatically
- nathanlee142
- 3 days ago
- 4 min read
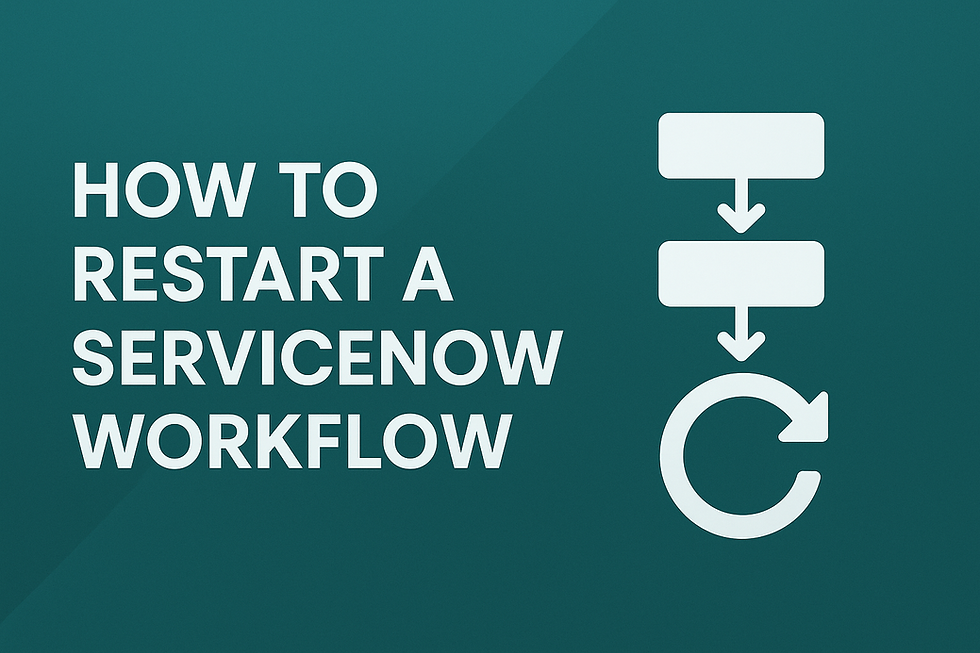
ServiceNow workflows are the backbone of many automated processes, guiding records through various stages. However, situations arise where an active workflow needs to be restarted from the beginning, perhaps due to incorrect initial data, a change in requirements, or a need to re-evaluate approvals. This article will guide you through the methods to programmatically restart a ServiceNow workflow, ensuring you can get your processes back on track when needed.
Introduction: Why Restart a ServiceNow Workflow?
Imagine a scenario where a user submits a request with incorrect information, triggering a complex approval workflow. Instead of manually intervening at each stage, the ability to restart the entire workflow from the beginning can save significant time and effort. Similarly, if business rules change mid-process, restarting the workflow ensures the record follows the updated logic. Understanding how to initiate a workflow restart via scripting is a valuable skill for any ServiceNow administrator or developer.
Main Body: Methods to Programmatically Restart a ServiceNow Workflow
ServiceNow provides a couple of effective ways to restart a workflow from a script, depending on whether you need to reset associated approvals.
Let's explore these methods:
1. Using the Workflow().restartWorkflow() Method
The most straightforward way to restart a workflow is by using the restartWorkflow() method available within the Workflow script include. This method takes two arguments:
current (GlideRecord): The GlideRecord object of the record for which you want to restart the workflow.
maintainStateFlag (boolean, optional): This boolean parameter determines whether existing approvals should be kept in their current state (true) or reset (false). If not specified, it defaults to true.
Step-by-Step Code Example:
Let's say you want to restart the workflow for a specific Incident record. You can use the following script:
// Get the sys_id of the incident record you want to restart the workflow for
var incidentSysId = 'YOUR_INCIDENT_SYS_ID'; // Replace with the actual sys_id
// Retrieve the incident record
var incident = new GlideRecord('incident');
if (incident.get(incidentSysId)) {
// Initialize the Workflow object
var wf = new Workflow();
// Restart the workflow, keeping existing approvals (maintainStateFlag defaults to true)
wf.restartWorkflow(incident);
gs.log('Workflow restarted for Incident: ' + incident.number + ' (approvals maintained).');
// To restart the workflow and reset approvals, use:
// wf.restartWorkflow(incident, false);
// gs.log('Workflow restarted for Incident: ' + incident.number + ' (approvals reset).');
} else {
gs.logError('Incident record with sys_id ' + incidentSysId + ' not found.');
}
Explanation:
We first obtain the sys_id of the target record. Remember to replace 'YOUR_INCIDENT_SYS_ID' with the actual sys_id.
We then retrieve the record using GlideRecord.
We instantiate a Workflow object.
We call the restartWorkflow() method, passing the GlideRecord object of the incident. By default, approvals will be maintained. To reset approvals, we pass false as the second argument.
Use Case: This method is useful when you need to rerun the workflow logic from the beginning without necessarily discarding existing approvals, for example, if the initial data has been corrected but existing approvals are still valid.
2. Using WorkflowApprovalUtils().cancelAll() followed by Workflow().restartWorkflow() for a Complete Restart
In scenarios where you need a clean restart, including the cancellation of all existing approvals, you can combine the cancelAll() method from the WorkflowApprovalUtils script include with the restartWorkflow() method.
Step-by-Step Code Example:
// Get the sys_id of the change request record you want to completely restart the workflow for
var changeRequestSysId = 'YOUR_CHANGE_REQUEST_SYS_ID'; // Replace with the actual sys_id
// Retrieve the change request record
var changeRequest = new GlideRecord('change_request');
if (changeRequest.get(changeRequestSysId)) {
// Initialize WorkflowApprovalUtils and Workflow objects
var wfa = new WorkflowApprovalUtils();
var wf = new Workflow();
// Cancel all existing approvals for the record
var comment = 'Workflow restarted programmatically, all previous approvals cancelled.';
wfa.cancelAll(changeRequest, comment);
// Restart the workflow, which will then generate new approvals
wf.restartWorkflow(changeRequest, false); // Setting maintainStateFlag to false for clarity
gs.log('Workflow completely restarted for Change Request: ' + changeRequest.number + ' (all approvals cancelled and reset).');
} else {
gs.logError('Change Request record with sys_id ' + changeRequestSysId + ' not found.');
}
Explanation:
Similar to the previous example, we get the record and initialize the necessary objects.
We then use wfa.cancelAll(changeRequest, comment) to cancel all active approvals related to the change request, adding a comment to the approval records.
Finally, we call wf.restartWorkflow(changeRequest, false) to restart the workflow and ensure that new approval activities are triggered from the beginning.
Use Case: This approach is ideal when you need to completely reset the workflow, including all approvals, perhaps because the initial conditions or data were fundamentally flawed.
Where to Use This Script
You can incorporate these scripts into various ServiceNow components, such as:
Business Rules: Trigger a workflow restart based on specific conditions or record updates.
Scripts - Background: For one-time or scheduled tasks that might require workflow restarts.
Workflow Script Activities: To programmatically restart a parent workflow or a sub-workflow based on certain criteria within the workflow itself.
Conclusion: Taking Control of Your ServiceNow Workflows
The ability to programmatically restart ServiceNow workflows provides you with greater control and flexibility in managing your automated processes. Whether you need a simple restart while preserving approvals or a complete reset including approvals, the Workflow().restartWorkflow() method, potentially combined with WorkflowApprovalUtils().cancelAll(), offers the solution. By implementing these techniques, you can effectively handle exceptions, adapt to changing requirements, and ensure your ServiceNow workflows function as intended from start to finish.