How to Retrieve All Users from a Group in ServiceNow using JavaScript
- nathanlee142
- Mar 20
- 3 min read
Updated: Mar 29
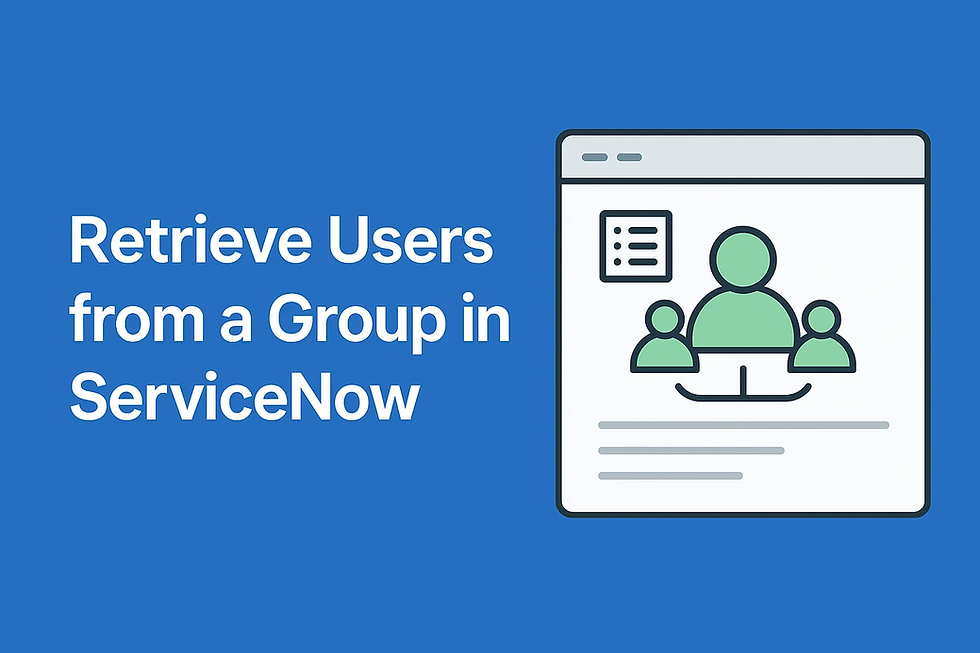
Managing user groups is crucial in ServiceNow, especially when automating processes, configuring notifications, or enhancing workflows. Developers often face scenarios where they need to obtain all users within a specific group programmatically. This article guides ServiceNow administrators and developers through a reliable method to fetch all users from a specific user group using JavaScript and GlideRecord.
Whether you're building a custom notification system, integrating with external applications, or automating incident assignments, accurately retrieving user details from groups is essential for efficient operations.
Understanding the Requirement and Common Issues
Let's start by clearly defining the scenario:
You have a group named "IS&T Senior Leadership Team," and you want to retrieve a list of all its members programmatically. Common reasons include populating notification lists, incident watchers, or integrating with external systems.
Common issues users encounter include retrieving incorrect data, confusion between user IDs and emails, or not correctly referencing the group members table (sys_user_grmember).
Step-by-Step Solution: Retrieve Users from a Group in ServiceNow
The recommended method involves using GlideRecord on the sys_user_grmember table, which holds records linking users to their groups.
Here is a clear, verified, and tested approach:
Step 1: Query the Group Members
First, perform a GlideRecord query on the sys_user_grmember table to fetch users based on group names:
function getUsersByGroup(groupName) {
var users = [];
// Find the group sys_id first
var grGroup = new GlideRecord('sys_user_group');
grGroup.addQuery('name', groupName);
grGroup.query();
if (grGroup.next()) {
var groupSysId = grGroup.getValue('sys_id');
var gr = new GlideRecord('sys_user_grmember');
gr.addQuery('group', groupSysId);
gr.query();
while (gr.next()) {
users.push(gr.getValue('user'));
}
}
return users; // returns array of user sys_ids
}
// Example usage:
var userSysIds = getUsersByGroup('IS&T Senior Leadership Team');
gs.info(userSysIds);
This function retrieves an array of user sys_ids, the unique identifiers for each user in ServiceNow.
Step 2: Fetching Additional User Information (User ID, Email, Name)
Often, just having the sys_id isn't sufficient. If you require additional details like the user ID, email address, or full name, you can enhance your script as follows:
function getDetailedUsersByGroup(groupName) {
var userDetails = [];
var groupGr = new GlideRecord('sys_user_group');
groupGr.addQuery('name', groupName);
groupGr.query();
if (!groupGr.next()) {
gs.error("Group '" + groupName + "' not found.");
return userDetails; // Return an empty array
}
var grMember = new GlideRecord('sys_user_grmember');
// Query using the group's sys_id
grMember.addQuery('group', groupGr.sys_id);
grMember.query();
while (grMember.next()) {
userDetails.push({
sys_id: grMember.user.sys_id.toString(),
user_name: grMember.user.user_name.toString(),
email: grMember.user.email.toString(),
full_name: grMember.user.name.toString()
});
}
return userDetails;
}
// Example usage:var seniorLeadershipUsers = getDetailedUsersByGroup('IS&T Senior Leadership Team');if (seniorLeadershipUsers.length > 0) {
seniorLeadershipUsers.forEach(function(user) {
gs.info("User ID: " + user.user_name + ", Email: " + user.email);
});
}
This approach uses "dot-walking" (navigating through references) to fetch details directly from the user records.
Practical Use Case Example: Updating a Watch List
Here's a common real-world scenario: automatically populating an incident's watch list with all users from a specific group.
// Business rule example (on Incident creation)
(function executeRule(current, previous /*null when async*/) {
var watchListGroup = 'IS&T Senior Leadership Team';
var users = getUsersByGroup(watchListGroup);
// watch_list expects comma-separated sys_ids
current.setValue('watch_list', users.join(','));
function getUsersByGroup(groupName) {
var userSysIds = [];
var gr = new GlideRecord('sys_user_grmember');
gr.addQuery('group.name', groupName);
gr.query();
while (gr.next()) {
userSysIds.push(gr.getValue('user'));
}
return userSysIds;
}
})(current, previous);
This automatically populates the incident watch list with members of the specified group, simplifying incident monitoring and improving team collaboration.
Alternative Approach: Out-of-the-Box Functionality
ServiceNow includes an out-of-the-box business rule (Report Helpers) with a predefined function (getGroupMembers) that can achieve similar results. Leveraging existing scripts is recommended to minimize customizations.
Conclusion
Accurately retrieving users from ServiceNow groups is straightforward and efficient using GlideRecord queries. By following the methods provided, administrators and developers can seamlessly integrate user group data into custom scripts, automation workflows, and notifications.
Actionable Next Steps for Developers:
Incorporate the provided GlideRecord approach into your scripts or business rules.
Test scripts in a non-production instance before deploying.
Consider leveraging existing ServiceNow business rules (Report Helpers) for built-in functionality.
With these approaches, you can confidently manage groups and users, enhancing the flexibility and efficiency of your ServiceNow environment.