Decoding ServiceNow Scripting: Why Your Array Push Might Not Be Working as Expected
- nathanlee142
- Mar 21
- 4 min read
Updated: Mar 30
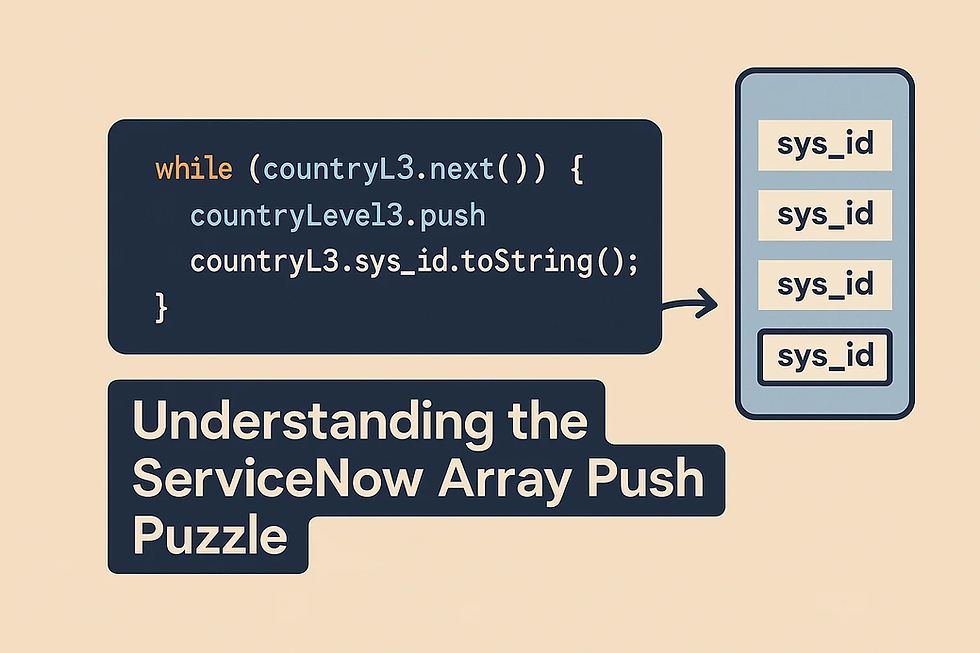
Are you a ServiceNow developer encountering unexpected behavior when trying to populate an array within a while loop? You're not alone! Many developers, especially those new to the platform or asynchronous JavaScript concepts, might stumble upon a peculiar issue where the push() method on an array seems to overwrite previous values instead of appending new ones. This article dives into this common scenario, explains why it happens in the context of ServiceNow's GlideRecord, and provides verified solutions to get your scripting back on track.
Understanding the ServiceNow Array Push Puzzle
Imagine you're writing a script to gather a list of related records in ServiceNow based on a certain condition. You might use a while loop in conjunction with a GlideRecord object to iterate through the matching records and add their unique identifiers (sys_ids) to an array. However, instead of a growing list of distinct sys_ids, you might find your array seemingly holding only the last processed value, or exhibiting other strange behaviors like the original poster experienced.
The initial code snippet from the ServiceNow community illustrates this problem perfectly:
JavaScript
var countryLevel3 =;
if (countryRef.u_organisation_level == 'Level 2') {
countryL3 = new GlideRecord('core_company');
countryL3.addQuery('u_reporting_level_2', countryRef.name);
countryL3.addQuery('u_organisation_level', 'Level 3');
countryL3.query();
while (countryL3.next()) {
countryLevel3.push(countryL3.sys_id);
// ... other logging ...
}
// ... further processing of countryLevel3 ...
}
The developer observed that despite the push() method being called in each iteration of the while loop, the countryLevel3 array didn't accumulate all the expected sys_ids. Instead, it appeared to be overwritten with the latest value in each cycle.
The Root Cause: Pushing References, Not Values
The key to understanding this behavior lies in how GlideRecord objects and their properties are handled in ServiceNow scripting. When you access countryL3.sys_id, you're not directly getting a simple string value. Instead, you're often interacting with a reference or a pointer to the sys_id property of the current GlideRecord object in the loop.
As the while loop iterates through the matching records, the countryL3 object moves to the next record. Consequently, the reference to countryL3.sys_id also updates to point to the sys_id of the new current record. When you push this reference into the array in each iteration, you're essentially storing multiple pointers that all ultimately point to the sys_id of the last GlideRecord object processed by the loop.
This explains why the original poster saw the array seemingly overwritten. By the time the loop finishes and the array is inspected, all the stored references are pointing to the final sys_id value encountered.
Verified Solutions: Ensuring You Push Actual Values
To correctly populate your array with the distinct sys_id values, you need to ensure that you are pushing the actual string value of the sys_id, not a reference. Here are a few verified solutions:
1. Using toString():
The most straightforward and commonly recommended solution is to explicitly convert the sys_id to a string using the toString() method:
JavaScript
countryLevel3.push(countryL3.sys_id.toString());
This method forces the retrieval of the current sys_id value as a string and pushes that string into the array.
2. Using getValue():
Another reliable approach is to use the getValue() method, which specifically retrieves the field value as a string:
JavaScript
countryLevel3.push(countryL3.getValue('sys_id'));
This method is generally considered good practice when you need to ensure you're working with the string representation of a field value from a GlideRecord.
3. Using getUniqueValue():
For retrieving the sys_id specifically, the getUniqueValue() method is also a valid option:
JavaScript
countryLevel3.push(countryL3.getUniqueValue());
This method is specifically designed to return the unique identifier of the current GlideRecord as a string.
Practical Example:
Let's revisit the original code snippet and apply the toString() solution:
JavaScript
var countryLevel3 =;
if (countryRef.u_organisation_level == 'Level 2') {
countryL3 = new GlideRecord('core_company');
countryL3.addQuery('u_reporting_level_2', countryRef.name);
countryL3.addQuery('u_organisation_level', 'Level 3');
countryL3.query();
while (countryL3.next()) {
countryLevel3.push(countryL3.sys_id.toString());
// Now countryLevel3 will correctly store each unique sys_id as a string
}
// ... further processing of countryLevel3 ...
}
By using toString(), getValue(), or getUniqueValue(), you ensure that a distinct string representation of the sys_id is added to the countryLevel3 array in each iteration of the while loop, resolving the overwriting issue.
Conclusion: Mastering GlideRecord Data Handling
Encountering unexpected behavior with array manipulation and GlideRecord objects is a common learning experience in ServiceNow development. The key takeaway from this scenario is the importance of understanding how GlideRecord properties are accessed and the distinction between references and actual values. By explicitly converting GlideRecord properties like sys_id to their string representations using methods like toString(), getValue(), or getUniqueValue(), you can reliably populate arrays and ensure your ServiceNow scripts function as intended.
Actionable Next Steps:
Whenever you need to store values from a GlideRecord object in an array, especially within a loop, remember to explicitly retrieve the value using .toString(), .getValue('fieldName'), or .getUniqueValue().
Pay close attention to the data types you are working with in your ServiceNow scripts. Understanding whether you are dealing with an object reference or a primitive value is crucial for avoiding unexpected outcomes.
Explore the ServiceNow documentation for GlideRecord to gain a deeper understanding of its methods and properties for efficient and accurate data manipulation.
By following these guidelines, you'll be well-equipped to handle array operations with GlideRecord objects effectively and build robust ServiceNow applications.